Leaderboard Implementation
Add a leaderboard to your game
Overview
Leaderboards list the top scores for the players who join an oooh hosted with your module. This fosters competition that starts at the oooh level, when a Creator decides to use your module to host a new oooh. The first players who join that oooh and submit their scores often find themselves coming back later to defend their spots on the leaderboard.
As more Hosts and their communities play your module, this competition blossoms into a global race where the best Oooh users in the world fight to prove they are the masters of the challenges offered by your module.
Leaderboards are especially powerful on Oooh, and they can be fundamental to a module's success. This page offers a getting started guide, code samples, and additional technical details that we hope you'll find useful while implementing excellent leaderboards in your modules.
Read our Design Primer too!
We'll cover the basics here, but we encourage you (and your designers) to check out our Leaderboard UX Guidelines page for more info about designing Oooh-worthy leaderboards.
Things You Should Know
Types of Leaderboards
Local Leaderboard
A local leaderboard is created for each oooh hosted using your module. This unique leaderboard is only accessible to everyone who joins that oooh.
Your module will be implementing views into the local leaderboard for ooohs hosted using your module and presenting them in your player experiences.
Global Leaderboard
An oooh's local leaderboard is separate from your module's global, Oooh-wide leaderboard. A global leaderboard is created for each Module on the Oooh platform. Scores submitted by your players will automatically be aggregated on Oooh's global leaderboard for your module.
Your module does not need to implement a view into the global leaderboard for your module.
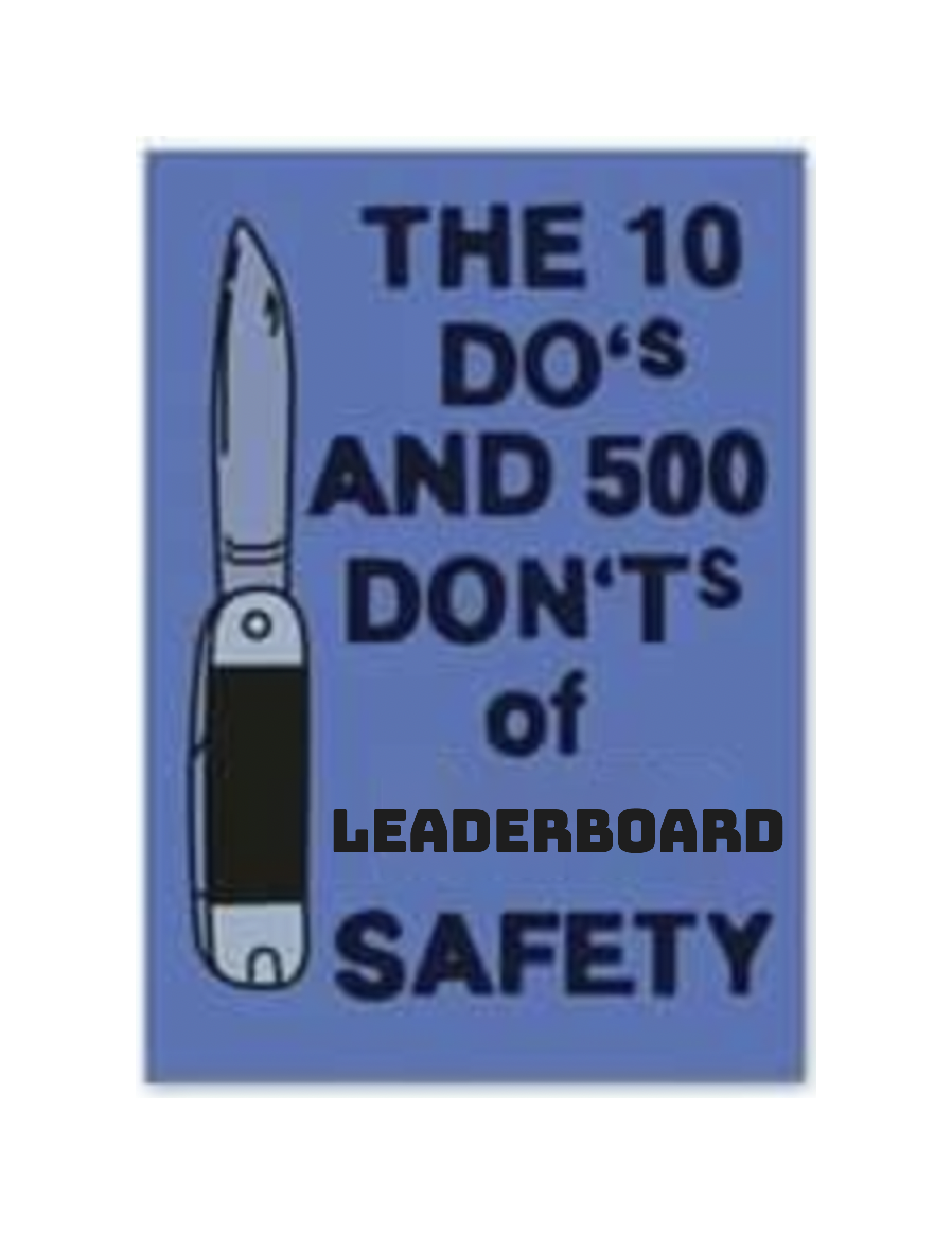
Always Submit Score on Module Complete
Whenever users finish your module, whether they're in the host or audience experience, you should call o3h.Instance.completeModule(FinalGameScore) to submit their score.
Requirements: Host Flow
In the Host Flow, creators use your module to record their ideal playthrough, including the initial replayData and score that will be presented to users who join the audience experience.
The typical Host flow looks like this:
- Show start screen
- Show any module-specific pre-game screen(s)
- Show gameplay screen
- Show any module-specific post-game screen(s)
- Show wrap-up screen (retry or end module)
- Submit the host's final score (when ending the module)
No Leaderboard in Host Flow
There should be no leaderboard present in your Host flow. The local leaderboard won't exist until after the host publishes the oooh, so do not call any of the leaderboard APIs either.
Handling Score
If hosts can earn a score in your module (most common), you should still keep track of the host's score and submit that score using o3h.Instance.completeModule(FinalGameScore) when they exit the module.
If your hosts don't earn a score in your module, submit this score with no parameters. We are working on better supporting this use case on the Platform.
Requirements: Audience Flow
Your module's audience experience should offer several views of the local leaderboard for players who join.
There are 3 types of leaderboard views that work well in the audience flow:
- Show start screen
- Show pre-game leaderboard screen (visually emphasize player's entry)
- Show any module-specific pre-game screen(s)
- Show gameplay screen, (including leaderboard chase, if applicable)
- Submit the player's score to the leaderboard
- Show post-game leaderboard screen, including score comparison and best achievement celebration (visually emphasize player's entry)
- Show any module-specific post-game screen(s)
- Show wrap-up screen (retry or end module)
The pre-game and post-game leaderboards should always show the top three scores, the host's original/replay score, and the current player's spot compared to these other entries.
This means a leaderboard in a module can display between 2 to 5 entries. See our Leaderboard UX Guidelines page for more info.
For more information on building a leaderboard chase experience, please get in touch.
Things to remember when implementing the Audience flow
- Do display a pre-game leaderboard
- Do track the player's final score and call UserDataService.addToLeaderboard at the end of the game. Do this every time before they can retry
- Do display a post-game leaderboard
- Do display the player's best achievement
- Do call o3h.Instance.completeModule(FinalGameScore) with the player's final score
Implementation Guide
LeaderboardData.js Wrapper Class
We recommend you use our LeaderboardData.js wrapper class when implementing the leaderboard in your module. This wrapper takes care of the logic for many use-cases (about 40) that leaderboards require.
If you're not able to use this wrapper, the rest of this guide should still be useful but please get in touch if you need additional support.
If you're able to use our wrapper, your studio only needs to handle displaying and animating the data that LeaderboardData.js returns.
Recipe: Using LeaderboardData.js
Check out the recipe below, which will walk you through using the LeaderboardData wrapper step by step.
Data Structure
Each LeaderboardData entry is structured as follows:
{
Rank: <number> This leaderboard entry's rank, 1-indexed. The best entry is rank 1.
Score: <number> This leaderboard entry's score
User: <see: module:o3h.UserDataService.UserInformation>
{
User.Name: <string> The display name of the user
User.AvatarImageUrl: <string> The Url of the image used for the avatar of the user
User.Level: <number> The integer value of the progression level of the user in the application
User.Type: <see: module:o3h.UserDataService.UserType> Which kind of user they are within the app
}
IsHost: <boolean Whether this is the host's entry when Oooh was created
IsHostReplay: <boolean> Whether this is the host's entry when joining their original Oooh
IsEmphasized: <boolean> Whether this entry should be visually emphasized
}
Types of LeaderboardData Entries
Standard Entry
Any player who finishes a game should be added to the leaderboard. This entry has its score updated every time a player beats their existing leaderboard score, so only one standard entry should exist per audience member.
Host Entries
Hosts can have up to 2 entries on the leaderboard for their oooh: their original entry and then a standard entry if they join their oooh as an audience member.
Hosts will submit their original entry through your module's host experience. This original leaderboard entry should be specially marked IsHost: true
. Oooh persists this entry so that the recorded video and reply data match for audience members who join.
Unlike standard entries, this score will never be overwritten.
However, Hosts have the ability to join their own ooohs again, and submit a score as an audience member. This second host entry is specially marked with IsHostReplay: true
, but otherwise functions the same as a standard leaderboard entry.
Updated over 2 years ago